JPEG Degrader Bake an image by recursively running JPEG compression on it (with filters).
Results
Summary
This project is a fun way to “bake” an image by recursively running JPEG compression on it (with filters) to create interesting effects.
Goals
My initial goal was to algorithmically take a jpeg and then compress it, and then compress it again and again while recording the process. I continued to play with it until I discovered that rotating the hue on each iteration caused the JPEG artifacts to behave like Conway’s Game of Life, or a reaction-diffusion system.
Solution
There’s 3 parts to this:
- Draw something to an HTML canvas.
- Generate a degraded JPEG image from the canvas.
- Copy the degraded image back to the canvas.
- Go to step 2.
With each iteration, we’ll get a more degraded image.
Suppose a setup like this:
<canvas id="canvas"></canvas>
<img id="img" />
<script>
const $canvas = document.getElementById("canvas");
const ctx = $canvas.getContext("2d");
const $img = document.getElementById("img");
</script>
We convert the canvas to an image like this:
$img.src = canvas.toDataURL("image/jpeg", 80);
And we draw the image back onto the canvas like so:
ctx.drawImage($img, 0, 0);
Do this enough times and we “bake” our image.
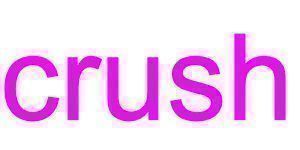
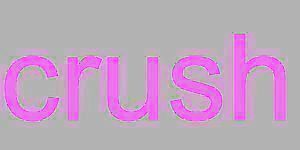
Because the browser APIs don’t have an option to provide sharpness, you won’t get the exact same effect. However, with a few modifications and tweaks, we can get some nice effects.
Add a few more tweaks and filters, and you get this:
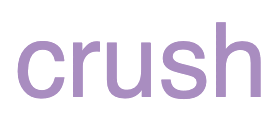
I used ezgif to shrink the GIFs and to further refine them (cropping, removing frames, looping, etc).